1 min to read
Create teams in Microsoft Teams through CSV using PowerShell
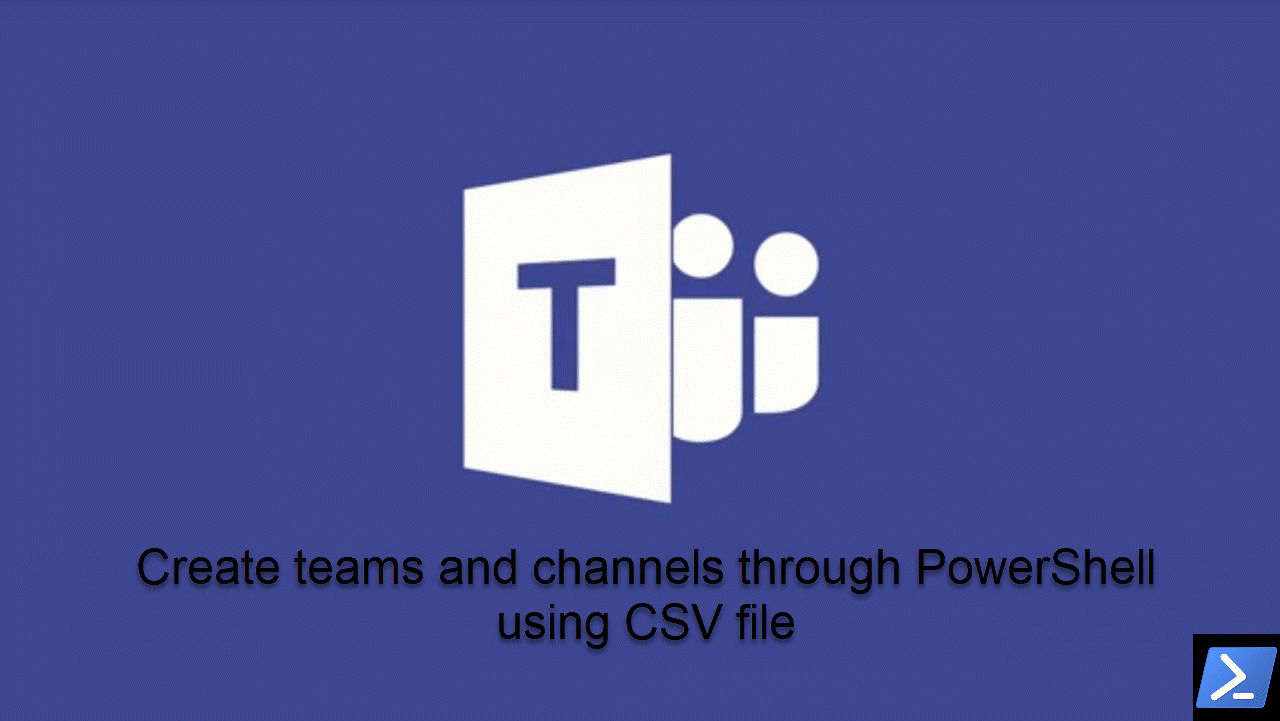
Using this PowerShell script you can create new teams with type, channels, owners and members in Microsoft Teams using csv file.
In the csv file used for team creation, you need to specify the following.
- New Team Name
- Team type (private or public)
- Channel names seperated by semicolon(;)
- Team Owner seperated by semicolon(;)
- Team Members seperated by semicolon(;)
If you want to create just team with General channel alone, don’t specify channel names, just leave it as empty.
function Create-Channel
{
param (
$ChannelName,$GroupId
)
Process
{
try
{
$teamchannels = $ChannelName -split ";"
if($teamchannels)
{
for($i =0; $i -le ($teamchannels.count - 1) ; $i++)
{
New-TeamChannel -GroupId $GroupId -DisplayName $teamchannels[$i]
}
}
}
Catch
{
}
}
}
function Add-Users
{
param(
$Users,$GroupId,$CurrentUsername,$Role
)
Process
{
try{
$teamusers = $Users -split ";"
if($teamusers)
{
for($j =0; $j -le ($teamusers.count - 1) ; $j++)
{
if($teamusers[$j] -ne $CurrentUsername)
{
Add-TeamUser -GroupId $GroupId -User $teamusers[$j] -Role $Role
}
}
}
}
Catch
{
}
}
}
function Create-NewTeam
{
param (
$ImportPath
)
Process
{
Import-Module MicrosoftTeams
$cred = Get-Credential
$username = $cred.UserName
Connect-MicrosoftTeams -Credential $cred
$teams = Import-Csv -Path $ImportPath
foreach($team in $teams)
{
$getteam= get-team |where-object { $_.displayname -eq $team.TeamsName}
If($getteam -eq $null)
{
Write-Host "Start creating the team: " $team.TeamsName
$group = New-Team -alias $team.TeamsName -displayname $team.TeamsName -AccessType $team.TeamType
Write-Host "Creating channels..."
Create-Channel -ChannelName $team.ChannelName -GroupId $group.GroupId
Write-Host "Adding team members..."
Add-Users -Users $team.Members -GroupId $group.GroupId -CurrentUsername $username -Role Member
Write-Host "Adding team owners..."
Add-Users -Users $team.Owners -GroupId $group.GroupId -CurrentUsername $username -Role Owner
Write-Host "Completed creating the team: " $team.TeamsName
$team=$null
}
}
}
}
Create-NewTeam -ImportPath "C:\Create team\NewTeams.csv"
CSV file format